Python 位元運算符用於對整數執行位元計算。 整數被轉換為二進制格式,然後逐位進行操作,因此稱為位元運算符。 Python 位元運算符僅對整數起作用,最終輸出以十進制格式返回。 Python 位元運算符也被稱為二進制運算符。
Python 位元運算符
Python 中有 6 個位元運算符。 下表提供了關於它們的簡短詳細信息。
Bitwise Operator | Description | Simple Example |
---|---|---|
& | Bitwise AND Operator | 10 & 7 = 2 |
Bitwise OR Operator | ||
^ | Bitwise XOR Operator | 10 ^ 7 = 13 |
~ | Bitwise Ones’ Compliment Operator | ~10 = -11 |
<< | Bitwise Left Shift operator | 10<<2 = 40 |
>> | Bitwise Right Shift Operator | 10>>1 = 5 |
讓我們逐一查看這些運算符,並了解它們的工作原理。
1. 位元 AND 運算符
如果兩個位元都是 1,則 Python 位元 and 運算符返回 1,否則返回 0。
>>> 10&7
2
>>>
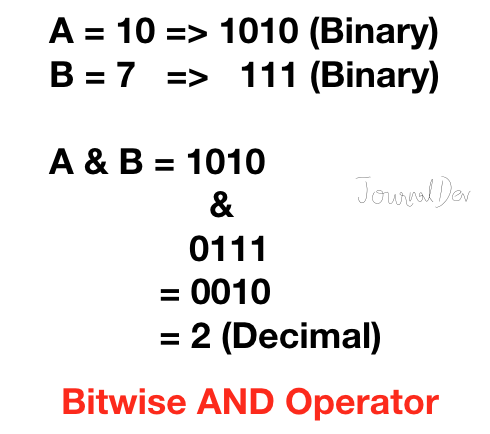
2. 位元 OR 運算符
如果任何一個位元是 1,則 Python 位元 or 運算符返回 1。 如果兩個位元都是 0,則返回 0。
>>> 10|7
15
>>>
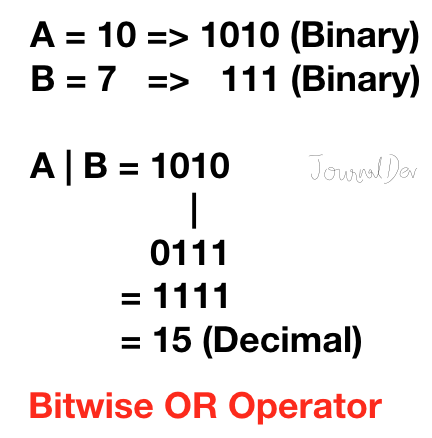
3. 位元 XOR 運算子
Python 的位元 XOR 運算子在其中一個位元為 0 而另一個位元為 1 時返回 1。如果兩個位元都為 0 或 1,則返回 0。
>>> 10^7
13
>>>
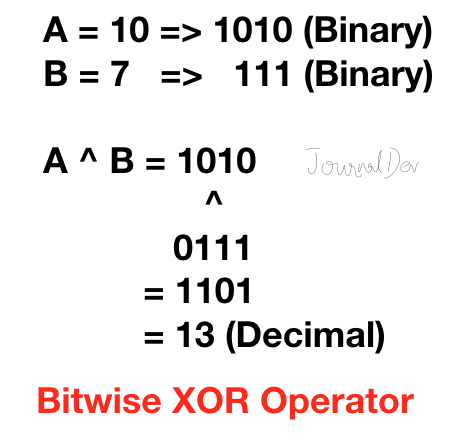
4. 位元 Ones’ 補數運算子
數字 ‘A’ 的 Ones’ 補數等於 -(A+1)。
>>> ~10
-11
>>> ~-10
9
>>>
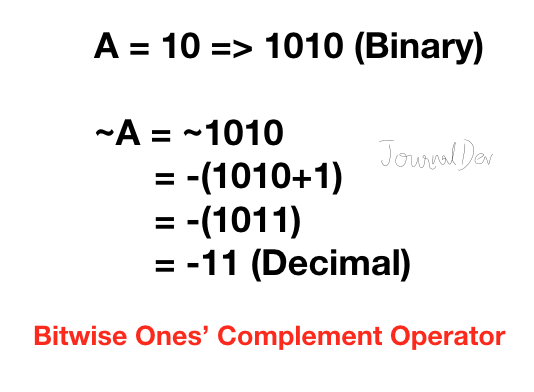
5. 位元左移運算子
Python 的位元左移運算子將左操作數的位元向左移動給定次數的右操作數。簡單來說,二進位數會在末尾添加 0。
>>> 10 << 2
40
>>>
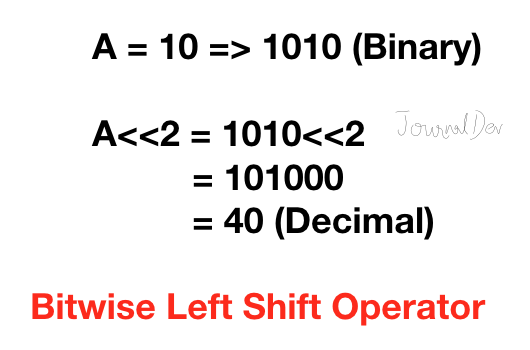
6. 位元右移運算子
Python 的右移運算子正好是左移運算子的相反。然後,左側操作數的位元將向右移動給定次數。簡單來說,右側的位元會被移除。
>>> 10 >> 2
2
>>>
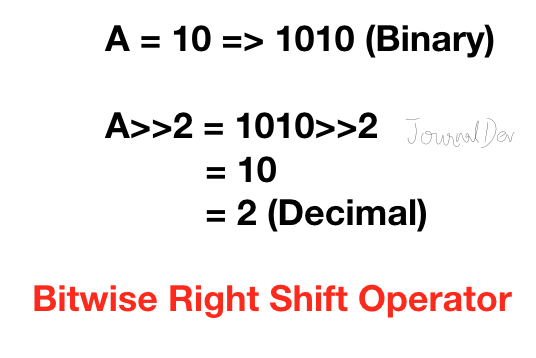
Python 位元運算子重載
Python 支援運算子重載。我們可以實現各種方法來支援我們自定義對象的位元運算子。
Bitwise Operator | Method to Implement |
---|---|
& | __and__(self, other) |
^ | __xor__(self, other) |
~ | __invert__(self) |
<< | __lshift__(self, other) |
>> | __rshift__(self, other) |
這是一個我們自定義對象的位元運算子重載的例子。
class Data:
id = 0
def __init__(self, i):
self.id = i
def __and__(self, other):
print('Bitwise AND operator overloaded')
if isinstance(other, Data):
return Data(self.id & other.id)
else:
raise ValueError('Argument must be object of Data')
def __or__(self, other):
print('Bitwise OR operator overloaded')
if isinstance(other, Data):
return Data(self.id | other.id)
else:
raise ValueError('Argument must be object of Data')
def __xor__(self, other):
print('Bitwise XOR operator overloaded')
if isinstance(other, Data):
return Data(self.id ^ other.id)
else:
raise ValueError('Argument must be object of Data')
def __lshift__(self, other):
print('Bitwise Left Shift operator overloaded')
if isinstance(other, int):
return Data(self.id << other)
else:
raise ValueError('Argument must be integer')
def __rshift__(self, other):
print('Bitwise Right Shift operator overloaded')
if isinstance(other, int):
return Data(self.id >> other)
else:
raise ValueError('Argument must be integer')
def __invert__(self):
print('Bitwise Ones Complement operator overloaded')
return Data(~self.id)
def __str__(self):
return f'Data[{self.id}]'
d1 = Data(10)
d2 = Data(7)
print(f'd1&d2 = {d1&d2}')
print(f'd1|d2 = {d1|d2}')
print(f'd1^d2 = {d1^d2}')
print(f'd1<<2 = {d1<<2}')
print(f'd1>>2 = {d1>>2}')
print(f'~d1 = {~d1}')
輸出:
Bitwise AND operator overloaded
d1&d2 = Data[2]
Bitwise OR operator overloaded
d1|d2 = Data[15]
Bitwise XOR operator overloaded
d1^d2 = Data[13]
Bitwise Left Shift operator overloaded
d1<<2 = Data[40]
Bitwise Right Shift operator overloaded
d1>>2 = Data[2]
Bitwise Ones Complement operator overloaded
~d1 = Data[-11]
如果您不熟悉新的字符串格式化,請閱讀 Python 中的 f-strings。
摘要
Python 位元運算子主要用於數學計算。我們也可以實現特定方法來支援自定義類實現的位元運算子。
Source:
https://www.digitalocean.com/community/tutorials/python-bitwise-operators